#import "YCHomeVC.h"
#import "YCHomeViewADCollectionCell.h"
#import "YCHomeViewVideoHeadCollectionCell.h"
#import "YCHomeViewVideoCollectionCell.h"
#import "YCHomeViewNewsHeadCollectionCell.h"
#import "YCHomeViewNewsCollectionCell.h"
@interface YCHomeVC ()<UICollectionViewDataSource,UICollectionViewDelegateFlowLayout>
@end
@implementation YCHomeVC
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
self.view.lqs_backgroundColor = CommonViewControllerBackgroundColor;
UICollectionViewFlowLayout *collectionLayout = [UICollectionViewFlowLayout new];
collectionLayout.sectionInset = UIEdgeInsetsMake(0, 15, 15, 15);
collectionLayout.minimumInteritemSpacing = 0;
// collectionLayout.minimumLineSpacing = 0;
UICollectionView *collectionView = [[UICollectionView alloc] initWithFrame:CGRectZero collectionViewLayout:collectionLayout];
collectionView.backgroundColor = [UIColor clearColor];
collectionView.dataSource = self;
collectionView.delegate = self;
[collectionView registerClass:[YCHomeViewADCollectionCell class] forCellWithReuseIdentifier:@"YCHomeViewADCollectionCell"];
[collectionView registerClass:[YCHomeViewVideoHeadCollectionCell class] forCellWithReuseIdentifier:@"YCHomeViewVideoHeadCollectionCell"];
[collectionView registerClass:[YCHomeViewVideoCollectionCell class] forCellWithReuseIdentifier:@"YCHomeViewVideoCollectionCell"];
[collectionView registerClass:[YCHomeViewNewsHeadCollectionCell class] forCellWithReuseIdentifier:@"YCHomeViewNewsHeadCollectionCell"];
[collectionView registerClass:[YCHomeViewNewsCollectionCell class] forCellWithReuseIdentifier:@"YCHomeViewNewsCollectionCell"];
[self lqs_boxControllerWithFillView:collectionView];
}
- (NSInteger) numberOfSectionsInCollectionView:(UICollectionView *)collectionView {
return 5;
}
- (NSInteger) collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
if (section == 0) {
return 1;
}
if (section == 1) {
return 1;
}
if (section == 2) {
return 2;
}
if (section == 3) {
return 1;
}
if (section == 4) {
return 5;
}
return 0;
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
if (indexPath.section == 0) {
if (indexPath.row == 0) {
YCHomeViewADCollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"YCHomeViewADCollectionCell" forIndexPath:indexPath];
return cell;
}
}
if (indexPath.section == 1) {
if (indexPath.row == 0) {
YCHomeViewVideoHeadCollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"YCHomeViewVideoHeadCollectionCell" forIndexPath:indexPath];
return cell;
}
}
if (indexPath.section == 2) {
YCHomeViewVideoCollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"YCHomeViewVideoCollectionCell" forIndexPath:indexPath];
return cell;
}
if (indexPath.section == 3) {
if (indexPath.row == 0) {
YCHomeViewNewsHeadCollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"YCHomeViewNewsHeadCollectionCell" forIndexPath:indexPath];
return cell;
}
}
if (indexPath.section == 4) {
YCHomeViewNewsCollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"YCHomeViewNewsCollectionCell" forIndexPath:indexPath];
return cell;
}
UICollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"UICollectionViewCell" forIndexPath:indexPath];
return cell;
}
- (CGSize) collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath {
if (indexPath.section == 0) {
if (indexPath.row == 0) {
CGFloat width = collectionView.frame.size.width - 15 - 15;
CGFloat height = [LQS heightWithWidth:width equalRatioWithOtherWidth:345 otherHeight:200];
return CGSizeMake(width, height);
}
}
if (indexPath.section == 1) {
if (indexPath.row == 0) {
CGFloat width = collectionView.frame.size.width - 15 - 15;
CGFloat height = 30;
return CGSizeMake(width, height);
}
}
if (indexPath.section == 2) {
CGFloat width = (collectionView.frame.size.width - 15 - 15 - 7) / 2;
CGFloat height = [LQS heightWithWidth:width equalRatioWithOtherWidth:169 otherHeight:105];
return CGSizeMake(width, height);
}
if (indexPath.section == 3) {
if (indexPath.row == 0) {
CGFloat width = collectionView.frame.size.width - 15 - 15;
CGFloat height = 30;
return CGSizeMake(width, height);
}
}
if (indexPath.section == 4) {
CGFloat width = collectionView.frame.size.width - 15 - 15;
CGFloat height = 90;
return CGSizeMake(width, height);
}
return CGSizeZero;
}
@end
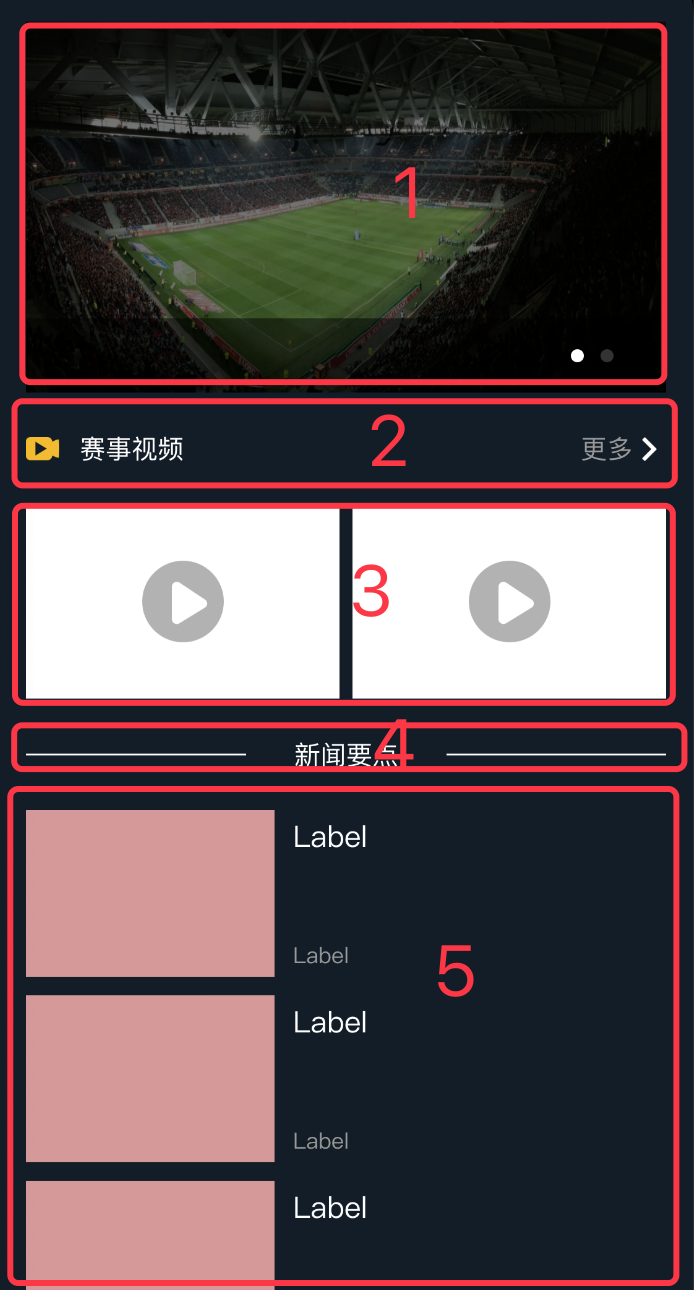