import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
home: new FirstScreen(),
);
}
}
class FirstScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text('第一个页面'),
backgroundColor: Colors.red,
),
body: new Center(
child: new RaisedButton(onPressed: (){
Navigator.push(context, new MaterialPageRoute(builder: (context) => new SecondScreen()),);
},
child: new Text('跳转'),),
),
);
}
}
class SecondScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text('第二个页面'),
backgroundColor: Colors.brown,
),
body: new Center(
child: new RaisedButton(onPressed: (){
Navigator.pop(context);
},
child: new Text('返回'),),
),
);
}
}
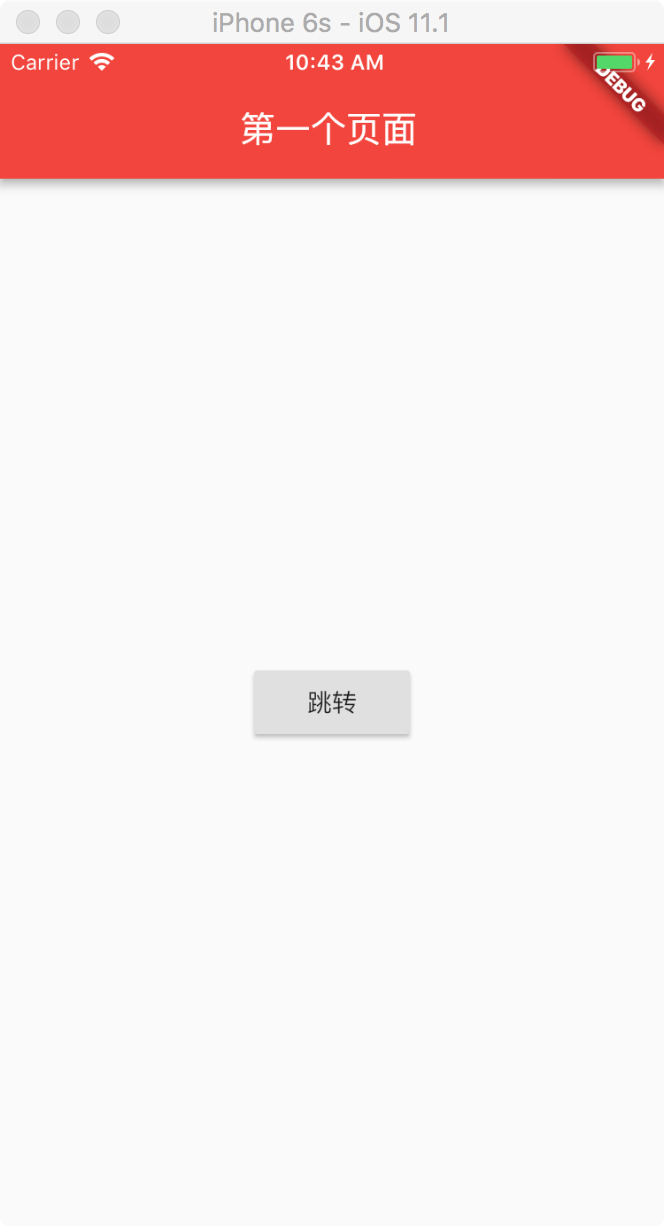
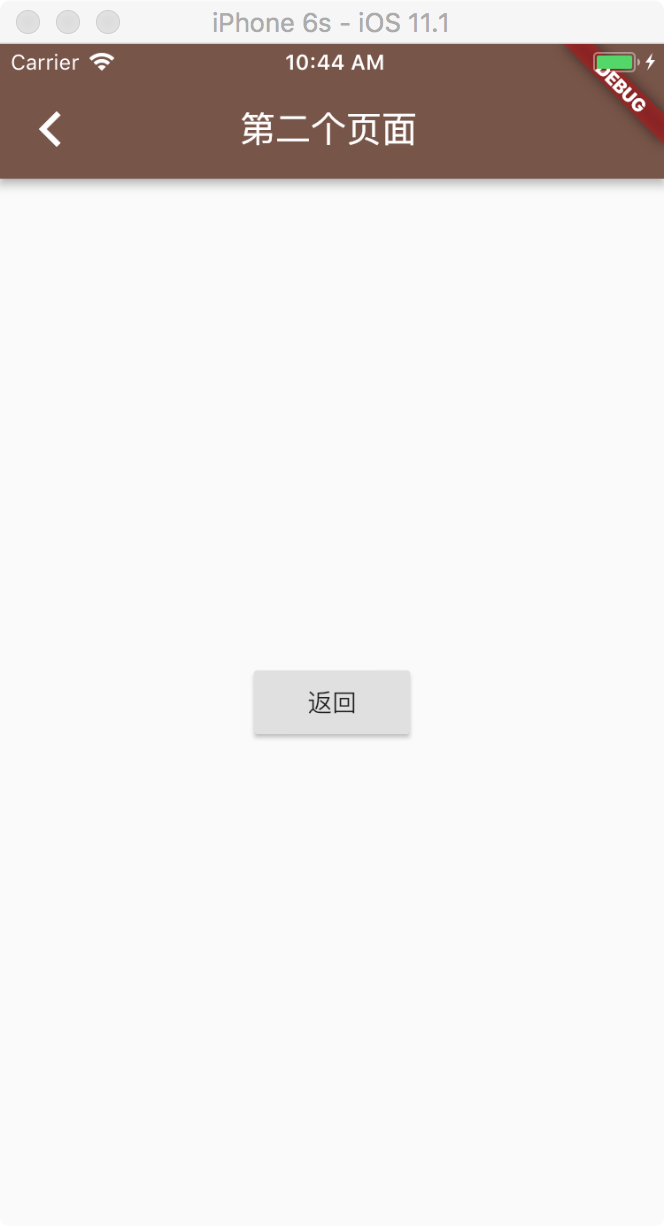