using System;
using Xamarin.Forms;
namespace one
{
public class TwoButtonPage : ContentPage
{
Button addButton, removeButton;
StackLayout loggerLayout = new StackLayout();
public TwoButtonPage()
{
addButton = new Button {
Text = "Add",
HorizontalOptions = LayoutOptions.CenterAndExpand
};
addButton.Clicked += OnButtonClicked;
removeButton = new Button {
Text = "Remove",
HorizontalOptions = LayoutOptions.CenterAndExpand,
IsEnabled = false
};
removeButton.Clicked += OnButtonClicked;
switch (Device.RuntimePlatform) {
case Device.iOS:
this.Padding = new Thickness(5, 20, 5, 5);
break;
}
this.Content = new StackLayout
{
Children = {
new StackLayout {
Orientation = StackOrientation.Horizontal,
Children = {
addButton,
removeButton
}
},
new ScrollView {
VerticalOptions = LayoutOptions.FillAndExpand,
Content = loggerLayout
}
}
};
}
void OnButtonClicked(object sender, EventArgs args) {
Button button = (Button)sender;
if (button == addButton) {
loggerLayout.Children.Add(new Label {
Text = "Button clicked at " + DateTime.Now.ToString("T")
});
} else {
loggerLayout.Children.RemoveAt(0);
}
removeButton.IsEnabled = loggerLayout.Children.Count > 0;
}
}
}
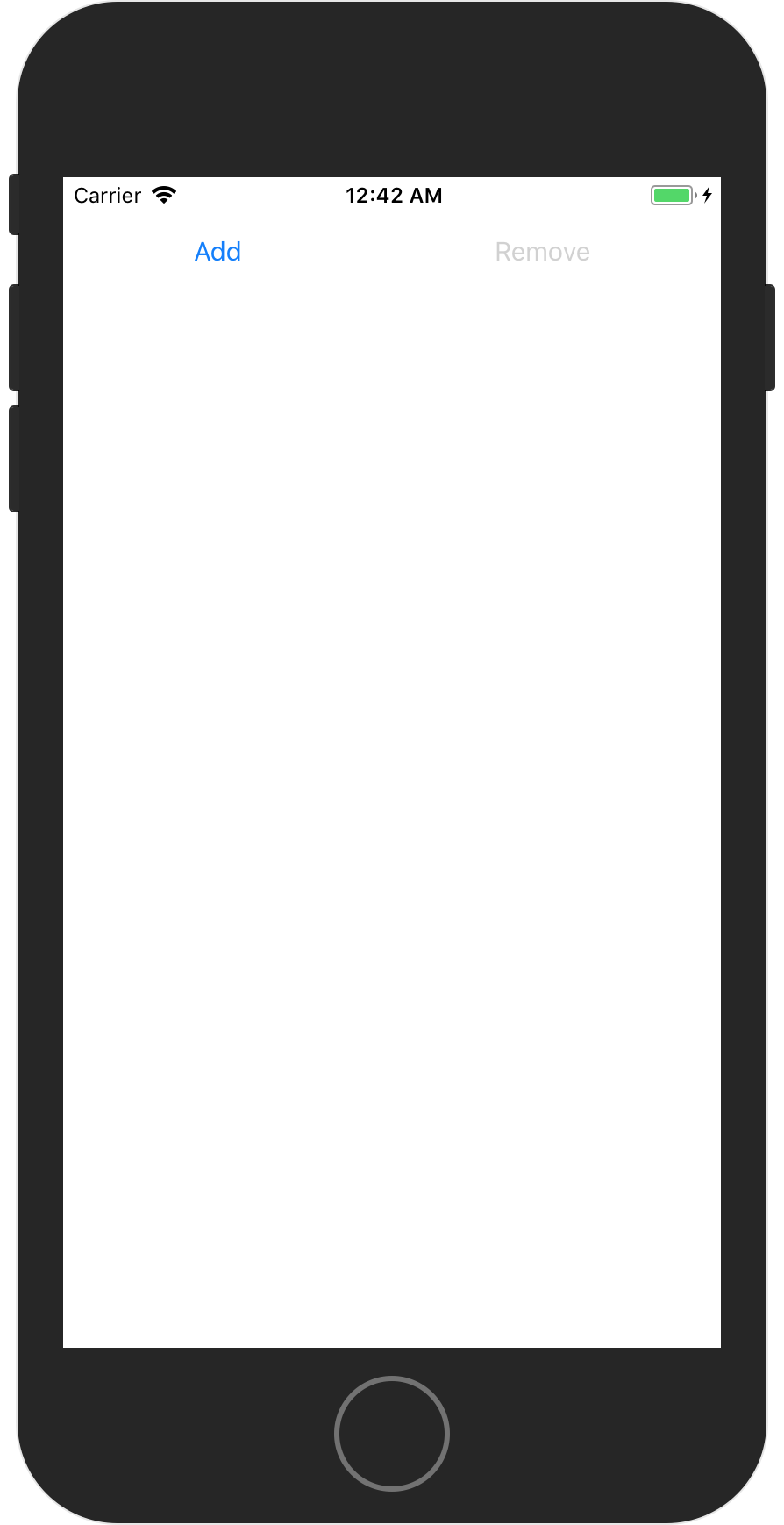
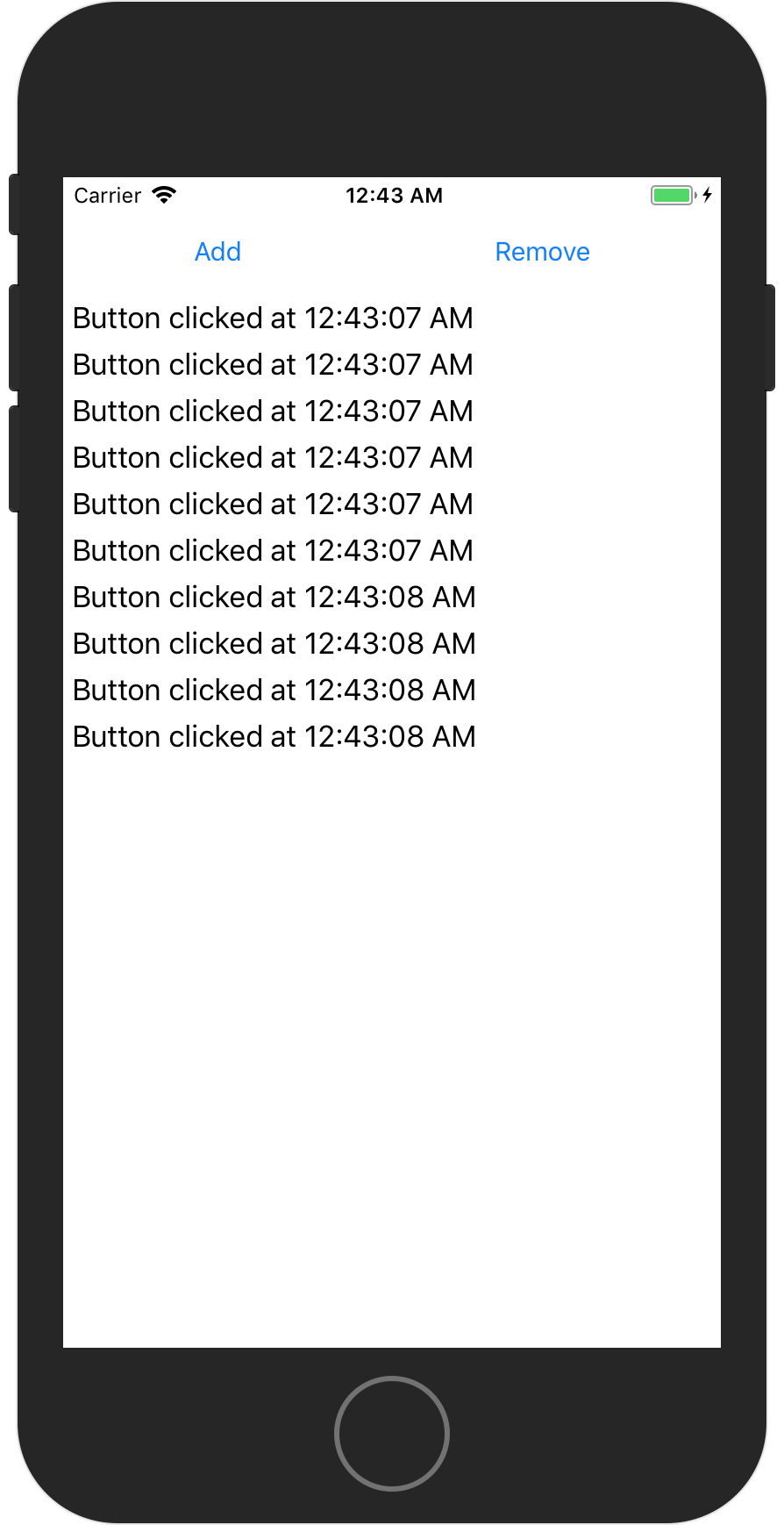